本文共 454 字,大约阅读时间需要 1 分钟。
1 MVC模型
- Model模型:数据模型和业务模型,数据模型用来存放业务数据,比如订单信息、用户信息等;而业务逻辑模型包含应用的业务操作,比如订单的添加或者修改等。
- View视图:要展示的数据,是一个广泛的概念,html,jsp,word,excel,json等。
- Controller控制器:控制应用程序的流程和处理用户所发出的请求。
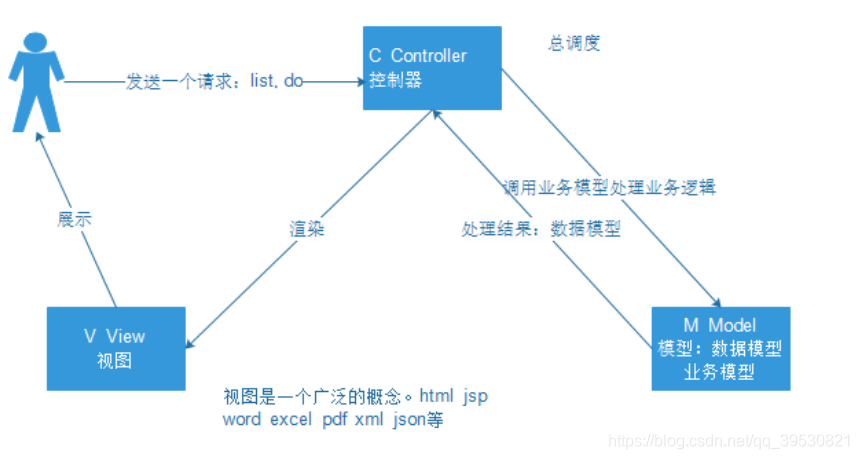
2 spring mvc介绍
- 大部分java应用是web应用,展现层是web应用最为重要的部分。spring为展现层提供了一个优秀的web框架springMVC。基于mvc设计理念,采用松散耦合可插拔组件接口,比其他mvn框架更具扩展性和灵活性。
- springMVC通过一套MVC主键,让pojo成为处理请求的控制器,无需实现任何接口,同时还支持REST风格的url请求。此外,在数据绑定,视图解析,本地化处理和静态资源处理上都有许多不俗的表现。
- springMVC围绕DispatcherServlet这个核心展开,负责截获请求并将其分派给相应的处理器处理。
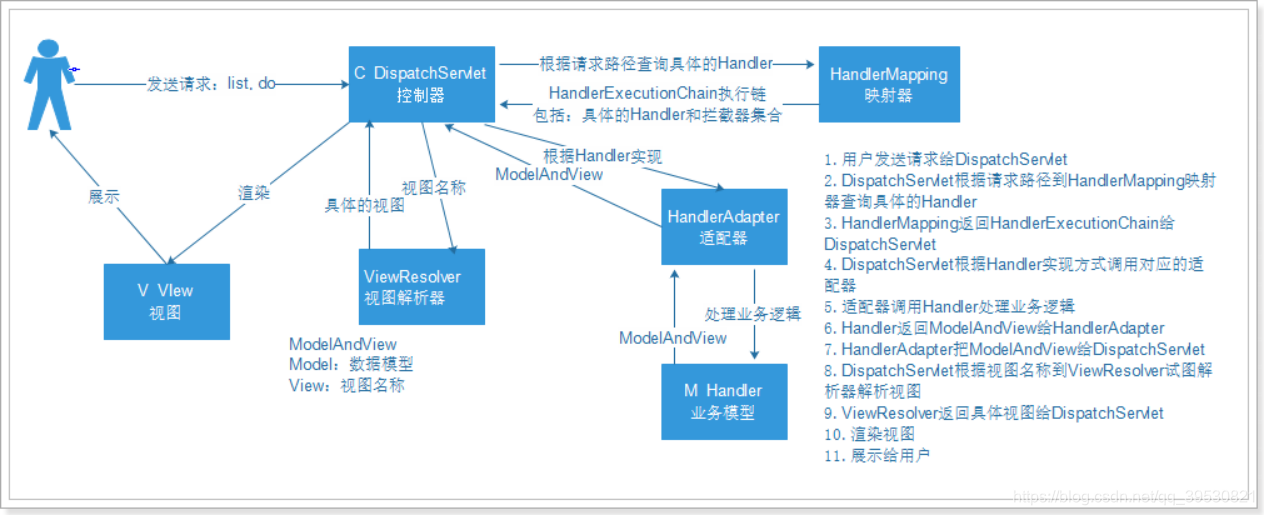
转载地址:http://gshwz.baihongyu.com/